Kuesa lights Example
Demonstrates the use of the KuesaSerenity API to manipulate light sources
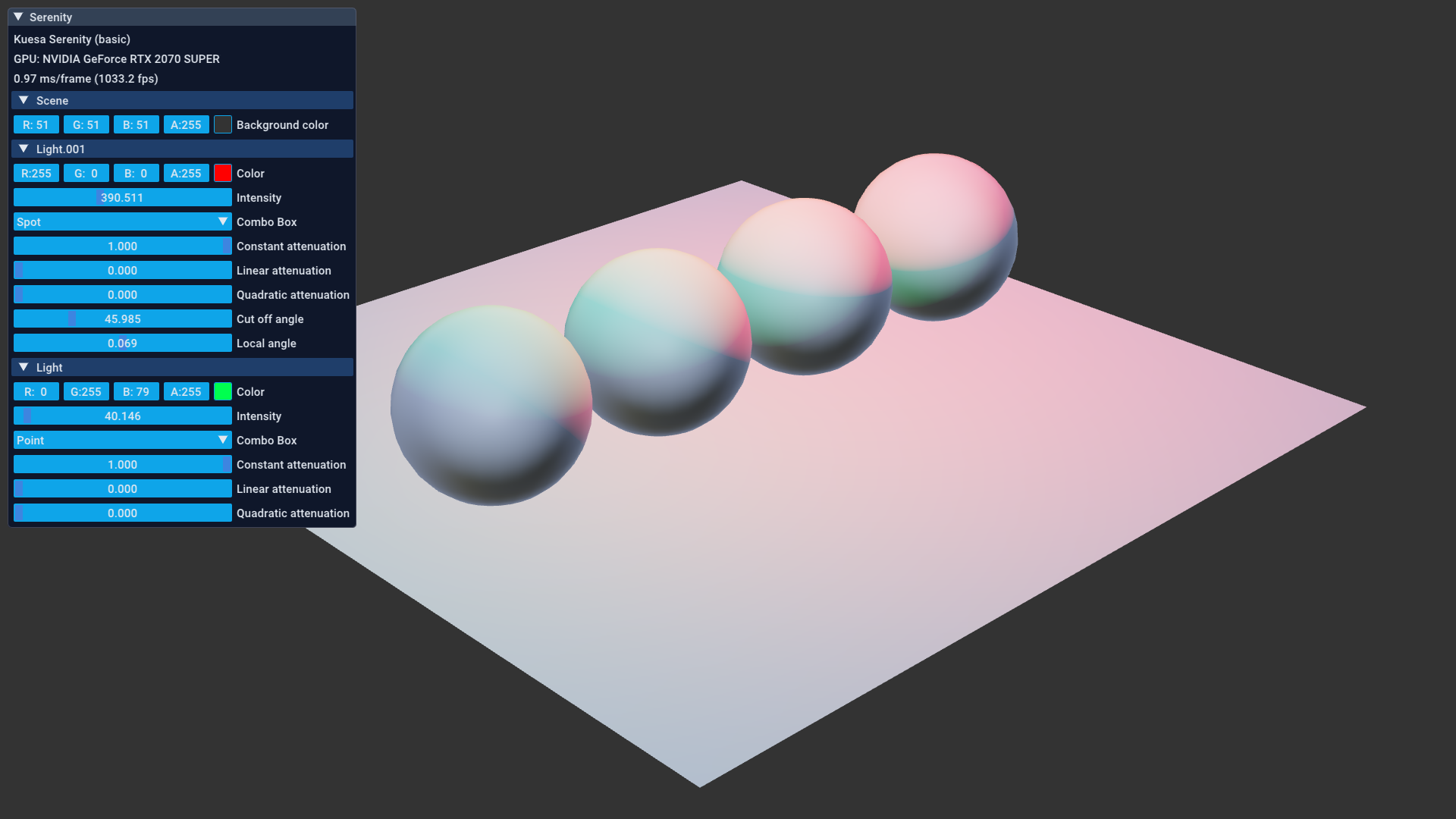
Setup Window
Kuesa::Serenity::Window can be conveniently used to create a Window suitable for Serenity.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15 | int main(int ac, char **av)
{
KDGui::GuiApplication app;
// Setup Window
Kuesa::Serenity::Window w;
auto engine = std::make_unique<::Serenity::AspectEngine>();
w.visible.valueChanged().connect([&app](const bool &visible) {
if (visible == false)
app.quit();
});
w.title = KDBindings::makeBinding(makeTitle(w.width, w.height, engine->fps));
w.width = 1920;
w.height = 1080;
w.visible = true;
|
Filename: lights-serenity/main.cpp
Importing a glTF2 File
We make use of the GLTF2Importer to load a glTF2 file and feed our AssectCollections . The file contains two light sources.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18 | std::unique_ptr<::Serenity::Entity> createKuesaScene(Kuesa::Serenity::AssetCollections *assetCollections,
::Serenity::LayerManager *layerManager)
{
Kuesa::Serenity::GLTF2Importer importer;
importer.assetCollections = assetCollections;
importer.layerManager = layerManager;
importer.source = String(SHARED_ASSETS_DIR "/models/lights/lights.gltf");
// Insert the generated Kuesa Entities into rootEntity
auto rootEntity = std::make_unique<::Serenity::Entity>();
for (std::unique_ptr<::Serenity::Entity> &sceneRoot : importer.sceneRoots()) {
// Take ownership
rootEntity->addChildEntity(std::move(sceneRoot));
}
return std::move(rootEntity);
}
|
Filename: lights-serenity/main.cpp
Manipulate the lights
The lights collection is then fed to the overlay code for display an manipulation.
| // ImGUI Overlay
Serenity::AbstractOverlay *overlay = createOverlay(w, engine.get(), algo.get(), collections.lights);
|
Filename: lights-serenity/main.cpp
The manipulation itself is done by setting each light property from each corresponding GUI element
| float intensity = light->intensity.get();
if (ImGui::SliderFloat(String("Intensity##" + name).c_str(), &intensity, 0, 1000))
light->intensity = intensity;
|
Filename: lights-serenity/main.cpp
Updated on 2023-07-03 at 11:02:17 +0000