Kuesa simple-cpp Example
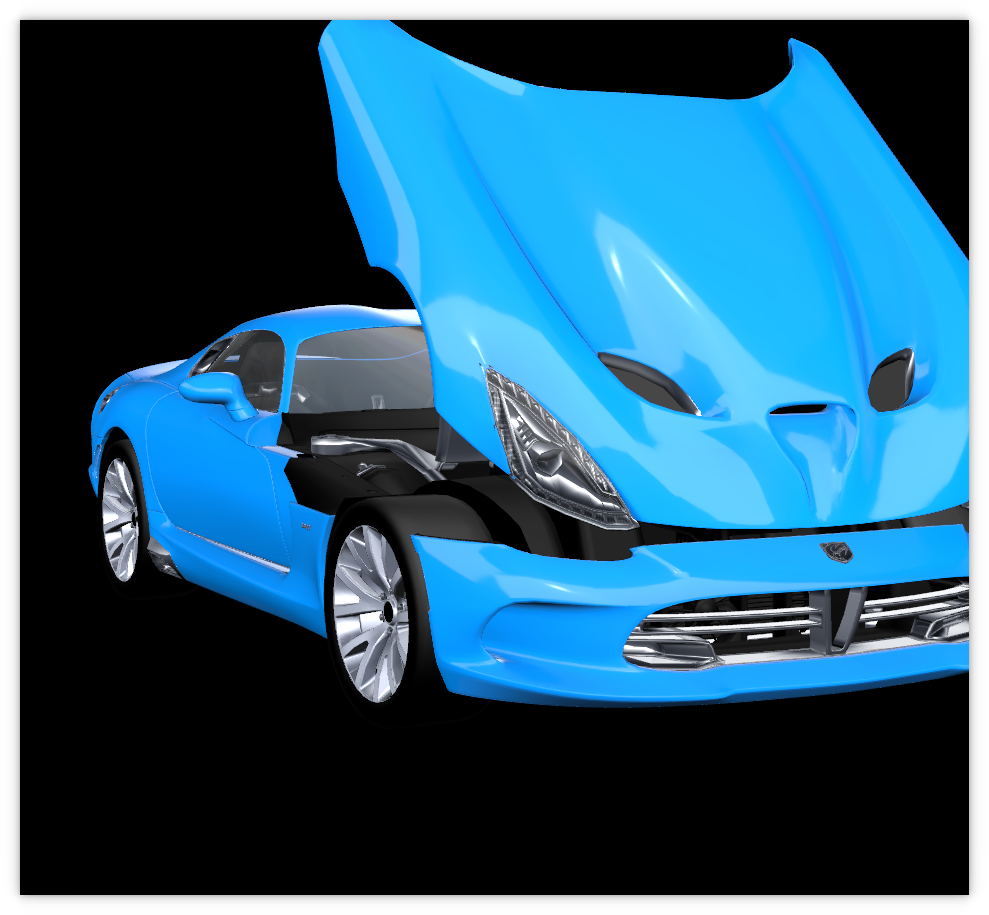
Setup Window and OpenGL requirements
setupDefaultSurfaceFormat can be conveniently used to request the appropriate OpenGL context requirements for Kuesa.
| int main(int ac, char **av)
{
// Set OpenGL requirements
Kuesa::Qt3D::setupDefaultSurfaceFormat();
|
Filename: simple-cpp/main.cpp
Next an instance of Window is created. It internally launches the Qt 3D engine and provides a wrapper around GLTF2Importer and ForwardRenderer .
| QGuiApplication app(ac, av);
KuesaUtils::Window view;
|
Filename: simple-cpp/main.cpp
Importing a glTF2 File
SceneConfiguration is used to speficy what glTF file to load and which animations to play. ViewConfiguration is used to specify from which camera the 3D scene should be viewed.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30 | KuesaUtils::SceneConfiguration *prepareScene()
{
KuesaUtils::SceneConfiguration *sceneConfiguration = new KuesaUtils::SceneConfiguration;
sceneConfiguration->setSource(QUrl("file:" ASSETS_DIR "/car.gltf"));
KuesaUtils::ViewConfiguration *mainViewConfiguration = new KuesaUtils::ViewConfiguration;
mainViewConfiguration->setCameraName(QStringLiteral("CamSweep_Orientation"));
sceneConfiguration->addViewConfiguration(mainViewConfiguration);
// List of animations to play
const QString animationNames[] = {
QStringLiteral("MatMotorBlockAction"),
QStringLiteral("TriggerMotorInfoAction"),
QStringLiteral("DoorLeftAction"),
QStringLiteral("DoorRightAction"),
QStringLiteral("HoodAction"),
QStringLiteral("SweepCamAction"),
QStringLiteral("SweepCamCenterAction"),
QStringLiteral("SweepCamPitchAction"),
};
for (const QString &animationName : animationNames) {
Kuesa::Qt3D::AnimationPlayer *player = new Kuesa::Qt3D::AnimationPlayer();
player->setClip(animationName);
player->setRunning(true);
player->setLoopCount(Kuesa::Qt3D::AnimationPlayer::Infinite);
sceneConfiguration->addAnimationPlayer(player);
}
return sceneConfiguration;
}
|
Filename: simple-cpp/main.cpp
| // Configure Scene Content
KuesaUtils::SceneConfiguration *sceneConfiguration = prepareScene();
// Set SceneConfiguration on 3D View
view.scene()->setActiveScene(sceneConfiguration);
// Show the window
view.show();
|
Filename: simple-cpp/main.cpp
Updated on 2022-10-18 at 11:12:52 +0200