Kuesa::Qt3D::OpacityMask
Module: Kuesa Qt 3D API
Masks onscreen content based on the alpha color value of a mask texture. More...
#include <opacitymask.h>
Inherits from Kuesa::Qt3D::AbstractPostProcessingEffect, Qt3DCore::QNode
Public Signals
Public Functions
Public Properties
Additional inherited members
Public Types inherited from Kuesa::Qt3D::AbstractPostProcessingEffect
Public Functions inherited from Kuesa::Qt3D::AbstractPostProcessingEffect
Protected Functions inherited from Kuesa::Qt3D::AbstractPostProcessingEffect
Protected Attributes inherited from Kuesa::Qt3D::AbstractPostProcessingEffect
Detailed Description
| class Kuesa::Qt3D::OpacityMask;
|
Masks onscreen content based on the alpha color value of a mask texture.
Given an RGBA mask texture, content of the backbuffer will be rendered as:
| vec4 pixelColor = vec4(inputColor.rgb, inputColor.a * maskColor.a)
|
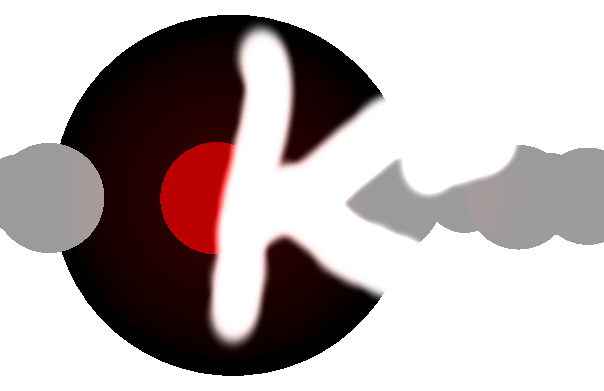
A premultiplied alpha variant of the algorithm is also available which can be of use when combining Qt 3D / Kuesa content with 2D QtQuick content (Scene3D). It performs rendering doing:
| vec4 pixelColor = vec4(inputColor.rgb / maskColor.a, inputColor.a * maskColor.a);
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19 | #include <Qt3DExtras/Qt3DWindow>
#include <ForwardRenderer>
#include <SceneEntity>
#include <OpacityMask>
Qt3DExtras::Qt3DWindow win;
Kuesa::Qt3D::SceneEntity *root = new Kuesa::Qt3D::SceneEntity();
Kuesa::Qt3D::ForwardRenderer *frameGraph = new Kuesa::Qt3D::ForwardRenderer();
Kuesa::Qt3D::OpacityMask *opacityMask = new Kuesa::Qt3D::OpacityMask();
Qt3DRender::QTextureLoader *texture = new Qt3DRender::QTextureLoader();
texture->setSource(QUrl("file:///opacityMask.png"));
opacityMask->setMask(texture);
frameGraph->addPostProcessingEffect(opacityMask);
win->setRootEntity(root);
win->setActiveFrameGraph(forwardRenderer);
...
|
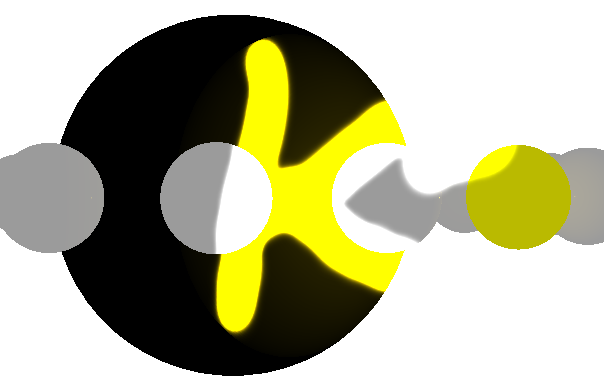
Public Signals Documentation
signal maskChanged
| void maskChanged(
Qt3DRender::QAbstractTexture * mask
)
|
signal premultipliedAlphaChanged
| void premultipliedAlphaChanged(
bool premultipliedAlpha
)
|
Public Functions Documentation
function OpacityMask
| explicit OpacityMask(
Qt3DCore::QNode * parent =nullptr
)
|
function frameGraphSubTree
| virtual FrameGraphNodePtr frameGraphSubTree() const override
|
Note: The lifetime of the returned subtree is assumed to be managed by the shared pointer. Any caller reparenting the subtree to add to a
Reimplements: Kuesa::Qt3D::AbstractPostProcessingEffect::frameGraphSubTree
Returns a FrameGraph subtree corresponding to the effect's implementation.
function layers
| virtual QVector< Qt3DRender::QLayer * > layers() const override
|
Reimplements: Kuesa::Qt3D::AbstractPostProcessingEffect::layers
Return the layers provided by this effect. This will generally be the layer of a
function setInputTexture
| virtual void setInputTexture(
Qt3DRender::QAbstractTexture * texture
) override
|
Reimplements: Kuesa::Qt3D::AbstractPostProcessingEffect::setInputTexture
Sets the input texture to texture for this effect. The texture contain the rendered scene that the effect will be applied to. This is set automatically by the ForwardRenderer when the effect is added.
function setMask
| void setMask(
Qt3DRender::QAbstractTexture * mask
)
|
function mask
| Qt3DRender::QAbstractTexture * mask() const
|
function setPremultipliedAlpha
| void setPremultipliedAlpha(
bool premultipliedAlpha
)
|
function premultipliedAlpha
| bool premultipliedAlpha() const
|
Public Property Documentation
property mask
| Qt3DRender::QAbstractTexture * mask;
|
The RGBA texture to use as a mask.
property premultipliedAlpha
Specifies whether the masking should be performed using premultipliedAlpha. This can be useful when combining Kuesa and QtQuick with a Scene3D element. It is false by default.
Updated on 2023-07-03 at 11:02:10 +0000